Expressions with Arrays
Expressions allow you to read values from the elements of an array. To get an elements value you must supply the name of the array, and the index of the element you want to read.
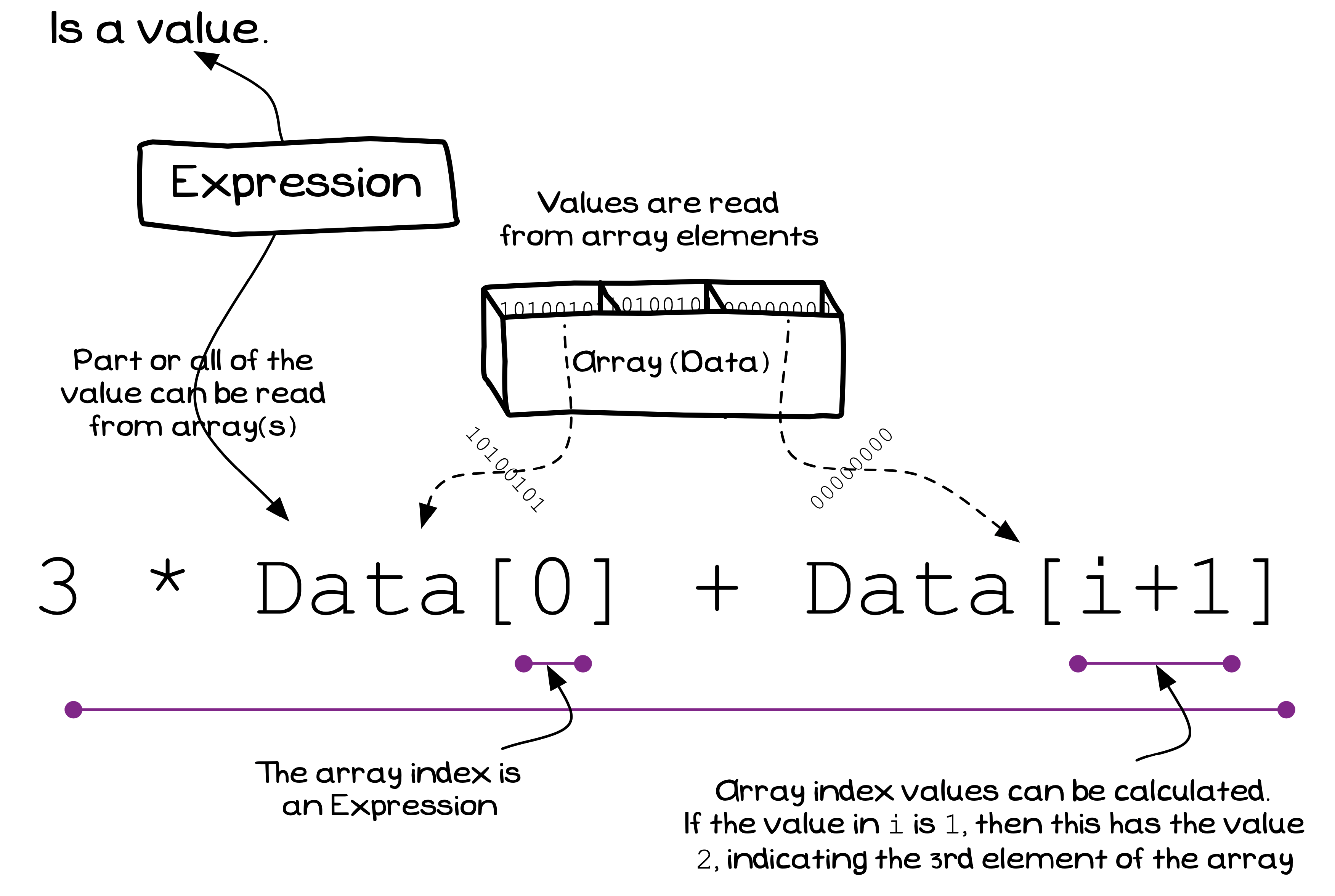
Reading Elements - Why, When, and How
In most ways, accessing an array element is the same as accessing a variable. The main difference, in terms of what you can do, is that you now have the index of the element you want to read as an expression. This is huge! Think about it. You can now have other code that works out which element to access. This is what makes arrays awesome!
Let’s think about life before arrays. Say I want to read in three integer values. I can do it like this:
#include <stdio.h>
int main(){ int data_0, data_1, data_2;
printf("Enter 3 integers (enter after each)"); scanf("%d", &data_0); scanf("%d", &data_1); scanf("%d", &data_2);
printf("You entered:"); printf("%d\n", data_0); printf("%d\n", data_1); printf("%d\n", data_2);
return 0;}
Each variable has a unique name, so we have lots of duplicate the code. With arrays, we can now change this up. To start with, we could code this with hard coded values. In this case we still have duplicate code, but you should be able to see that arrays are much like a number of variables used to store the same thing.
#include <stdio.h>
int main(){ const int NUM_VALUES = 3; int data[NUM_VALUES];
printf("Enter %d integers (enter after each)", NUM_VALUES);
scanf("%d", &data[0]); scanf("%d", &data[1]); scanf("%d", &data[2]);
printf("You entered:"); printf("%d\n", data[0]); printf("%d\n", data[1]); printf("%d\n", data[2]);
return 0;}
Where it becomes cool, is where we realise that the value for the index can be calculated! It can be any expression we want at all! As a first step, we could remove the hard coded indexes.
#include <stdio.h>
int main(){ const int NUM_VALUES = 3; int data[NUM_VALUES]; int i;
printf("Enter %d integers (press 'Enter' after each value)", NUM_VALUES);
i = 0; scanf("%d", &data[i]); i++; scanf("%d", &data[i]); i++; scanf("%d", &data[i]);
printf("You entered:"); i = 0; printf("%d\n", data[i]); i++; printf("%d\n", data[i]); i++ printf("%d\n", data[i]);
return 0;}
Now the code is screaming out to you to remove the repetition. We are performing exactly the same steps over and over here.
#include <stdio.h>
int main(){ const int NUM_VALUES = 3; int data[NUM_VALUES]; int i;
printf("Enter %d integers (enter after each)", NUM_VALUES);
i = 0; while ( i < NUM_VALUES ) { scanf("%d", &data[i]); i++; }
printf("You entered:"); i = 0; while ( i < NUM_VALUES ) { printf("%d\n", data[i]); i++; }
return 0;}
We can make this neater using for loops, but I hope you can see the potential here.
How do we now change this from working with 3 values to work with 50, 100, 1000, 10000 values? Easy! You just change the NUM_VALUES
constant. That is all.
Think about it. You now have the tools to work with large numbers of values, and it doesn’t take you much more to code this than it takes to code the actions for one value. You just add a loop, and the computer does all the hard work repeating those steps over and over for all the values you give it.